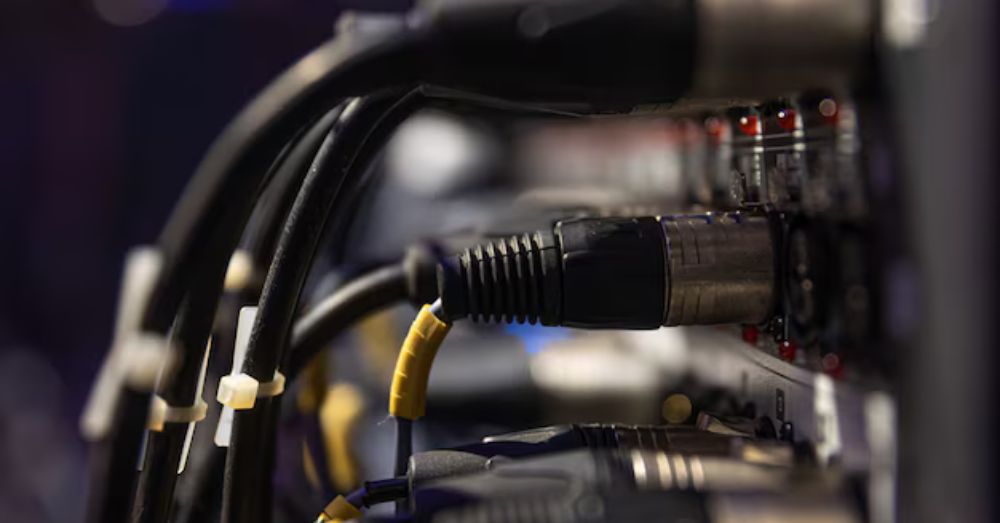
Wowza Gradle
Introduction
Wowza Gradle brings together two powerful technologies: Wowza Streaming Engine, a widely used media server, and Wowza Gradle, a versatile build automation tool. In the realm of live streaming, having an efficient development environment is critical for seamless deployment, maintenance, and scaling of streaming solutions. By integrating Wowza Streaming Engine with Wowza Gradle, developers can automate builds, streamline deployments, and manage dependencies more effectively.
This article explores the key concepts, use cases, setup process, and best practices for using Wowza Gradle, providing a detailed roadmap for developers aiming to improve their streaming applications.
What is Wowza Streaming Engine?
Wowza Streaming Engine is a high-performance media server used to deliver live and on-demand audio, video, and other streaming content to various devices. It offers robust support for multiple protocols, such as:
- RTMP (Real-Time Messaging Protocol)
- HLS (HTTP Live Streaming)
- DASH (Dynamic Adaptive Streaming over HTTP)
- WebRTC (Real-Time Communication)
Wowza Streaming Engine is designed for reliability and scalability, supporting professional-grade streaming services for enterprises, broadcasters, and educational institutions.
Key Features of Wowza Streaming Engine
- Adaptive bitrate streaming: Automatically adjusts the video quality according to the viewer’s network conditions.
- Low latency: Supports WebRTC and CMAF for low-latency streaming.
- Flexible protocols: Delivers content via multiple streaming formats.
- Extensible architecture: Allows developers to extend functionalities with Java modules.
- Cloud-based and on-premises deployment: Offers flexibility to run on the cloud or local infrastructure.
What is Gradle?
Gradle is an open-source build automation tool used primarily for Java, Android, and Groovy-based projects. It is known for its flexibility, high performance, and ability to manage complex build configurations. Gradle allows developers to define how their code is compiled, packaged, and deployed through build scripts written in Groovy or Kotlin DSL (Domain-Specific Language).
Why Use Gradle?
- Dependency management: Wowza Gradle makes it easy to manage project dependencies through repositories such as Maven Central and JCenter.
- Build automation: Automates compiling, testing, packaging, and deployment of code.
- Plugin support: Provides a wide range of plugins for various tasks (e.g., Java, Kotlin, Docker).
- Incremental builds: Optimizes build time by only rebuilding parts of the project that have changed.
- Custom tasks: Developers can define their own tasks to extend Wowza Gradle’s functionality.
Why Integrate Wowza Streaming Engine with Gradle?
Using Wowza Gradle together offers several advantages, particularly for development and continuous integration (CI/CD) workflows. The integration ensures faster builds, smoother deployments, and better version control of custom Wowza modules.
Benefits of Wowza Gradle Integration
- Automated builds: Automate the process of compiling and packaging Wowza custom modules.
- Dependency management: Easily manage third-party libraries or modules required for streaming functionality.
- Continuous integration: Integrate Wowza development into a CI/CD pipeline to ensure frequent and reliable deployments.
- Custom tasks: Define Wowza Gradle tasks to control Wowza server operations, such as restarting or deploying configurations.
- Versioning and rollback: Manage different versions of Wowza modules and roll back changes if necessary.
Setting Up Wowza Gradle Integration
Integrating Wowza Streaming Engine with Wowza Gradle involves setting up both tools and creating a build environment where custom modules can be compiled and deployed automatically. Below is a step-by-step guide to setting up the integration.
Prerequisites
Before you begin, ensure the following:
- Wowza Streaming Engine is installed and running on your machine.
- Java Development Kit (JDK) is installed (version 8 or later).
- Gradle is installed and added to your system path.
- IDE (e.g., IntelliJ, Eclipse) for coding.
- Internet access to download dependencies.
Step 1: Install and Configure Wowza Streaming Engine
- Download Wowza Streaming Engine from the Wowza website.
- Install the server by following the instructions for your operating system.
- Start Wowza Streaming Engine and access the management console (usually available at
http://localhost:8088
). - Create a new Application in Wowza to test your custom module.
Step 2: Create a Wowza Gradle Project
Open a terminal or command prompt and create a new Gradle project by running the following command:
bashCopy codegradle init --type java-application
This command generates the necessary files for a Java-based Gradle project, including the build.gradle
file, which is the core configuration file for Gradle builds.
Step 3: Configure build.gradle
for Wowza Modules
Edit the build.gradle
file to include Wowza-specific dependencies and configurations. Add the following:
groovyCopy codeplugins {
id 'java'
}
group 'com.example'
version '1.0.0'
repositories {
mavenCentral()
}
dependencies {
// Include Wowza's core library for custom module development
implementation 'com.wowza:wse-lib:4.8.0' // Adjust version as needed
}
// Define custom task to deploy the Wowza module
task deployModule(type: Copy) {
from 'build/libs'
into '/path/to/wowza/lib'
}
This configuration includes the Wowza core library as a dependency and defines a custom deployModule
task to copy the compiled module into Wowza’s lib
directory.
Step 4: Develop a Custom Wowza Module
Create a Java class for your custom Wowza module. Below is a simple example of a Wowza module that logs when a stream starts.
javaCopy codepackage com.example.wowza;
import com.wowza.wms.module.ModuleBase;
import com.wowza.wms.stream.IMediaStream;
public class MyWowzaModule extends ModuleBase {
public void onStreamCreate(IMediaStream stream) {
getLogger().info("Stream started: " + stream.getName());
}
}
Place this class in the src/main/java/com/example/wowza
directory within your Gradle project.
Step 5: Build the Wowza Module
To compile the module, run the following command from the project’s root directory:
bashCopy codegradle build
This command compiles the Java code and packages it into a JAR file located in the build/libs
directory.
Step 6: Deploy the Module to Wowza
Use the custom deployModule
task defined in the build.gradle
file to deploy the module:
bashCopy codegradle deployModule
Alternatively, you can manually copy the JAR file to the Wowza lib
directory.
Step 7: Restart Wowza Streaming Engine
After deploying the module, restart the Wowza server for the changes to take effect. You can restart it from the Wowza management console or using the following command:
bashCopy codesudo service WowzaStreamingEngine restart
Automating Builds and Deployment with CI/CD
For large-scale projects, integrating Wowza and Gradle into a continuous integration pipeline can enhance efficiency. Here’s how to do it with Jenkins, a popular CI tool.
Step 1: Install Jenkins
Install Jenkins on your server and configure it to monitor your code repository (e.g., GitHub or GitLab).
Step 2: Create a Jenkins Pipeline
Create a Jenkins pipeline that automates the following steps:
- Clone the repository.
- Run
gradle build
to compile the code. - Execute
gradle deployModule
to deploy the module to Wowza. - Restart Wowza Streaming Engine.
Example Jenkinsfile:
groovyCopy codepipeline {
agent any
stages {
stage('Build') {
steps {
sh 'gradle build'
}
}
stage('Deploy') {
steps {
sh 'gradle deployModule'
}
}
stage('Restart Wowza') {
steps {
sh 'sudo service WowzaStreamingEngine restart'
}
}
}
}
Best Practices for Wowza and Gradle Integration
- Modular design: Break your Wowza modules into smaller, reusable components.
- Version control: Use versioning in your Gradle project to track module changes.
- Testing: Write unit tests for your custom modules to ensure functionality.
- Error handling: Implement proper logging and error handling in Wowza modules.
- Backup configurations: Always back up Wowza configuration files before deploying new modules.
Troubleshooting Common Issues
- Dependency conflicts: Ensure that Wowza Gradle dependencies do not conflict with Wowza’s internal libraries.
- Module not loading: Verify that the module JAR is in the correct directory and restart Wowza.
- Gradle build failures: Check for syntax errors or missing dependencies in your
build.gradle
file. - Permissions issue: Ensure your user account has the necessary permissions to deploy and restart Wowza.
Conclusion
Integrating Wowza Streaming Engine with Wowza Gradle offers developers a powerful toolset for building, deploying, and managing custom streaming modules efficiently. With the right setup, you can streamline your development process, automate deployments, and scale your streaming services with ease. By following the steps and best practices outlined in this article, you can unlock the full potential of Wow